Python의 Tutle 그래픽을 이용해 재미 있는 미니 게임을 만드는 방법에 대해서 알아보도록 하겠습니다. Turtle은 초보자들도 쉽게 그래픽을 다룰 수 있게 해주는 Python의 내장 모듈 입니다.
Turtle 모듈 소개
Turtle 그래픽은 화면상의 '거북이'를 움직여 그림을 그리는 방식으로 작동 합니다. 간단한 명령어로 복잡한 그래픽을 만들 수 있어 프로그래밍 입문자들이게 인기가 많습니다.
import turtle
screen = turtle.Screen()
t = turtle.Turtle()
이렇게 Turtle을 import하고 screen과 turle 객체를 생서하는 것으로 시작 합니다.
간단한 Snake 게임 만들기
이제 Turtle을 이용해 고전 게임인 Snake를 만들어보겠습니다.
1.게임 화면 설정
screen.setup(600, 600)
screen.bgcolor("black")
screen.title("Snake Game")
이 코드로 600x600 크기의 검은색 게임 화면을 만듭니다.
2.뱀 만들기
snake = turtle.Turtle()
snake.shape("square")
snake.color("white")
snake.penup()
snake.goto(0, 0)
snake.direction = "stop"
뱀을 표현할 Turtle 객체를 만들고 초기 설정을 합니다.
3.먹이 만들기
food = turtle.Turtle()
food.shape("circle")
food.color("red")
food.penup()
food.goto(0, 100)
뱀이 먹을 먹이도 Turtle 객체로 만듭니다.
4.뱀 이동 함수 만들기
def move():
if snake.direction == "up":
y = snake.ycor()
snake.sety(y + 20)
elif snake.direction == "down":
y = snake.ycor()
snake.sety(y - 20)
elif snake.direction == "left":
x = snake.xcor()
snake.setx(x - 20)
elif snake.direction == "right":
x = snake.xcor()
snake.setx(x + 20)
이 함수로 뱀의 이동을 구현 합니다.
5.키보드 입력 처리
def go_up():
if snake.direction != "down":
snake.direction = "up"
def go_down():
if snake.direction != "up":
snake.direction = "down"
def go_left():
if snake.direction != "right":
snake.direction = "left"
def go_right():
if snake.direction != "left":
snake.direction = "right"
screen.listen()
screen.onkeypress(go_up, "w")
screen.onkeypress(go_down, "s")
screen.onkeypress(go_left, "a")
screen.onkeypress(go_right, "d")
키보드 입력을 받아 뱀의 방향을 바꾸는 함수들을 정의 합니다.
6.게임 루프 만들기
while True:
screen.update()
if snake.distance(food) < 20:
x = random.randint(-290, 290)
y = random.randint(-290, 290)
food.goto(x, y)
move()
time.sleep(0.1)
이 루프에서 게임의 주요 로직이 실행됩니다. 뱀이 먹이를 먹으면 먹이의 위치가 바뀌고, 뱀은 계속 이동합니다.
게임 개선하기
이제 기본적인 Snake 게임이 완성되었습니다. 여기에 몇 가지 기능을 추가해 게임을 더 재미있게 만들어 봅시다.
1.점수 시스템 추가
score = 0
high_score = 0
score_display = turtle.Turtle()
score_display.speed(0)
score_display.color("white")
score_display.penup()
score_display.hideturtle()
score_display.goto(0, 260)
score_display.write(f"Score: {score} High Score: {high_score}", align="center", font=("Courier", 24, "normal"))
이 코드로 화면상단에 점수를 표시할 수 있습니다.
2.뱀 길이 늘리기
segments = []
# 게임 루프 내에서
if snake.distance(food) < 20:
x = random.randint(-290, 290)
y = random.randint(-290, 290)
food.goto(x, y)
new_segment = turtle.Turtle()
new_segment.speed(0)
new_segment.shape("square")
new_segment.color("grey")
new_segment.penup()
segments.append(new_segment)
score += 10
if score > high_score:
high_score = score
score_display.clear()
score_display.write(f"Score: {score} High Score: {high_score}", align="center", font=("Courier", 24, "normal"))
이 코드를 추가하면 뱀이 먹이를 먹을 때마다 길이가 늘어나고 점수가 올라갑니다.
3.게임 오버 조건 추가
if snake.xcor() > 290 or snake.xcor() < -290 or snake.ycor() > 290 or snake.ycor() < -290:
time.sleep(1)
snake.goto(0, 0)
snake.direction = "stop"
for segment in segments:
segment.goto(1000, 1000)
segments.clear()
score = 0
score_display.clear()
score_display.write(f"Score: {score} High Score: {high_score}", align="center", font=("Courier", 24, "normal"))
이 코드로 뱀이 화면 경계에 부딪히면 게임이 재시작됩니다.
완벽한 코드
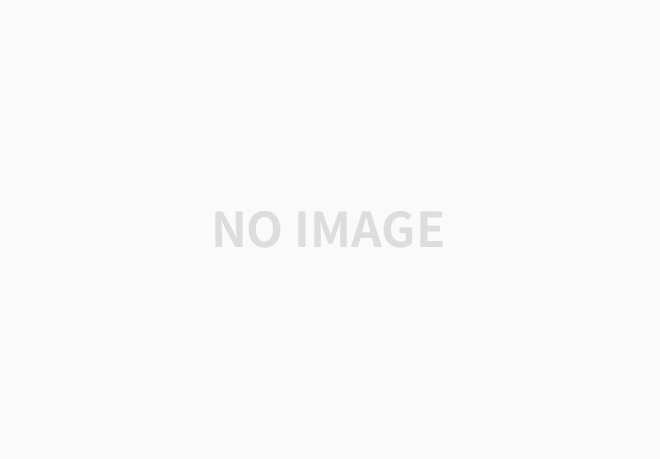
import turtle
import time
import random
# 화면 설정
screen = turtle.Screen()
screen.setup(600, 600)
screen.bgcolor("black")
screen.title("Snake Game")
screen.tracer(0)
# 뱀 만들기
snake = turtle.Turtle()
snake.shape("square")
snake.color("white")
snake.penup()
snake.goto(0, 0)
snake.direction = "stop"
# 먹이 만들기
food = turtle.Turtle()
food.shape("circle")
food.color("red")
food.penup()
food.goto(0, 100)
# 점수 표시
score = 0
high_score = 0
score_display = turtle.Turtle()
score_display.speed(0)
score_display.color("white")
score_display.penup()
score_display.hideturtle()
score_display.goto(0, 260)
score_display.write(f"Score: {score} High Score: {high_score}", align="center", font=("Courier", 24, "normal"))
# 뱀 몸통 세그먼트 리스트
segments = []
# 뱀 이동 함수
def move():
if snake.direction == "up":
y = snake.ycor()
snake.sety(y + 20)
elif snake.direction == "down":
y = snake.ycor()
snake.sety(y - 20)
elif snake.direction == "left":
x = snake.xcor()
snake.setx(x - 20)
elif snake.direction == "right":
x = snake.xcor()
snake.setx(x + 20)
# 방향 전환 함수
def go_up():
if snake.direction != "down":
snake.direction = "up"
def go_down():
if snake.direction != "up":
snake.direction = "down"
def go_left():
if snake.direction != "right":
snake.direction = "left"
def go_right():
if snake.direction != "left":
snake.direction = "right"
# 키보드 바인딩
screen.listen()
screen.onkeypress(go_up, "w")
screen.onkeypress(go_down, "s")
screen.onkeypress(go_left, "a")
screen.onkeypress(go_right, "d")
# 게임 메인 루프
while True:
screen.update()
# 벽과 충돌 체크
if snake.xcor() > 290 or snake.xcor() < -290 or snake.ycor() > 290 or snake.ycor() < -290:
time.sleep(1)
snake.goto(0, 0)
snake.direction = "stop"
for segment in segments:
segment.goto(1000, 1000)
segments.clear()
score = 0
score_display.clear()
score_display.write(f"Score: {score} High Score: {high_score}", align="center", font=("Courier", 24, "normal"))
# 먹이 먹기
if snake.distance(food) < 20:
x = random.randint(-290, 290)
y = random.randint(-290, 290)
food.goto(x, y)
new_segment = turtle.Turtle()
new_segment.speed(0)
new_segment.shape("square")
new_segment.color("grey")
new_segment.penup()
segments.append(new_segment)
score += 10
if score > high_score:
high_score = score
score_display.clear()
score_display.write(f"Score: {score} High Score: {high_score}", align="center", font=("Courier", 24, "normal"))
# 뱀 몸통 이동
for index in range(len(segments)-1, 0, -1):
x = segments[index-1].xcor()
y = segments[index-1].ycor()
segments[index].goto(x, y)
if len(segments) > 0:
x = snake.xcor()
y = snake.ycor()
segments[0].goto(x, y)
move()
# 자기 몸과 충돌 체크
for segment in segments:
if segment.distance(snake) < 20:
time.sleep(1)
snake.goto(0, 0)
snake.direction = "stop"
for segment in segments:
segment.goto(1000, 1000)
segments.clear()
score = 0
score_display.clear()
score_display.write(f"Score: {score} High Score: {high_score}", align="center", font=("Courier", 24, "normal"))
time.sleep(0.1)
# 게임 종료 (이 부분은 실행되지 않지만, 필요시 screen.exitonclick()을 사용할 수 있습니다)
screen.mainloop()
마무리
이렇게 해서 Turtle 그래픽을 이용한 간단한 Snake 게임을 만들어 보았습니다. 이 기본 구조를 바탕으로 더 많은 기능을 추가하거나 다른 종류의 게임을 만들어볼 수 있습니다. 예를 들어, 장애물을 추가하거나, 레벨 시스템을 구현하거나, 두 명이서 플레이할 수 있는 모드를 만들어볼 수 있겠죠.
Turtle 그래픽은 간단하면서도 강력한 도구입니다. 이를 통해 프로그래밍의 기본 개념들을 재미있게 학습할 수 있습니다. 여러분만의 창의적인 아이디어로 더 흥미로운 게임을 만들어보세요!
'IT > Python' 카테고리의 다른 글
[Python] 자연어 처리 기초: NLTK 라이브러리 활용하기 (0) | 2025.01.20 |
---|---|
[Python] TensorFlow를 이용한 딥러닝 모델 구현하기 (0) | 2025.01.20 |
[Python] 파이썬을 이용한 퍼즐 게임 구현하기 (0) | 2025.01.19 |
[Python] 파이썬으로 텍스트 기반 RPG 게임 개발하기 (0) | 2025.01.19 |
[Python] Pygame으로 간단한 2D 게임 만들기 (0) | 2025.01.19 |